Company Website for Metalbau Kausch with PayloadCMS
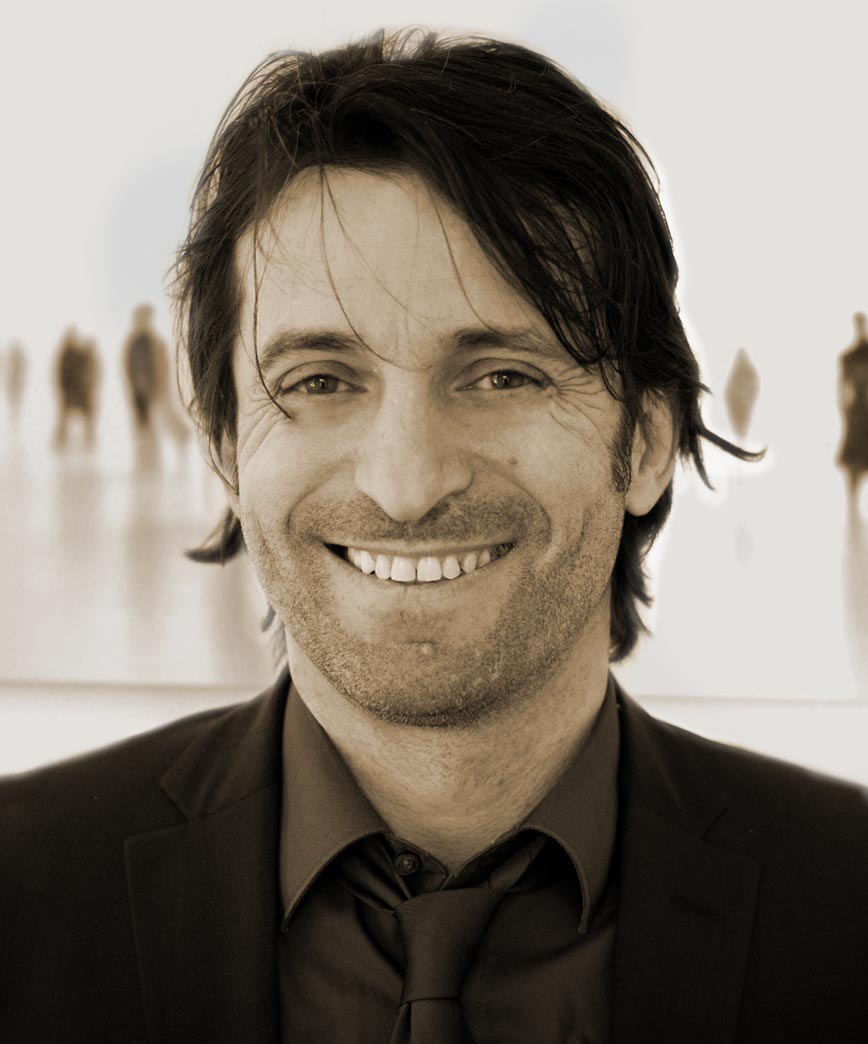
- Published on
- Duration
- 3 Months
- Role
- Front- & Back-End Developer, UI-Designer
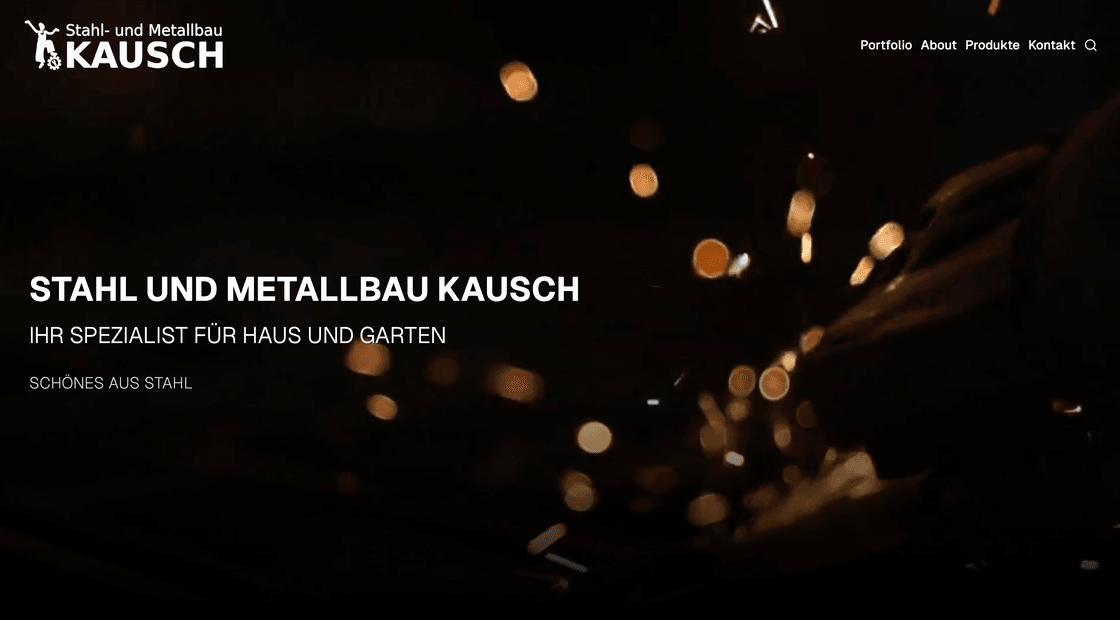
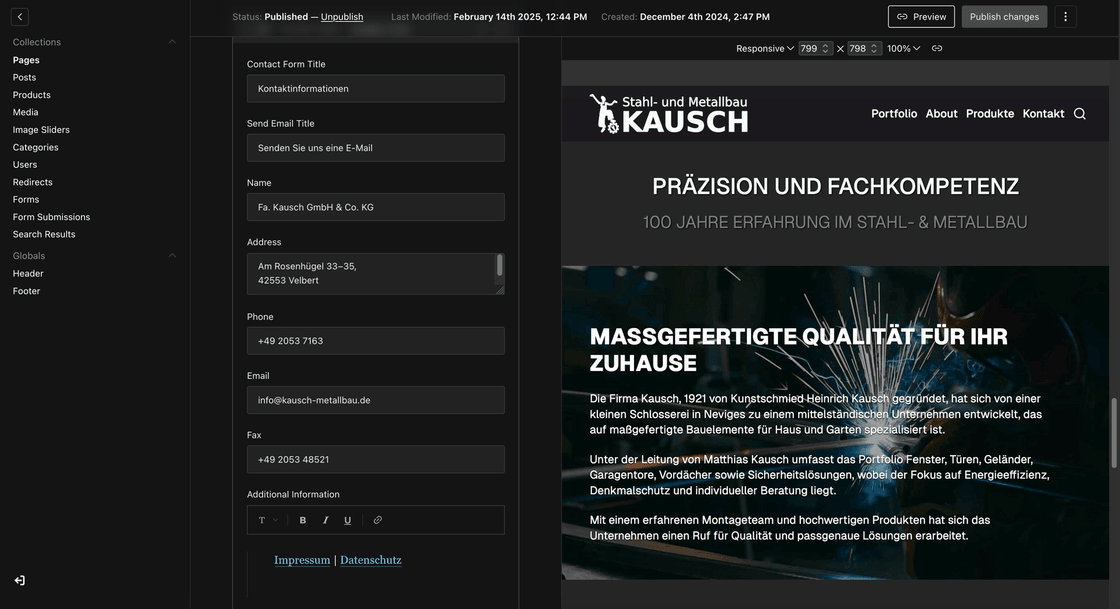
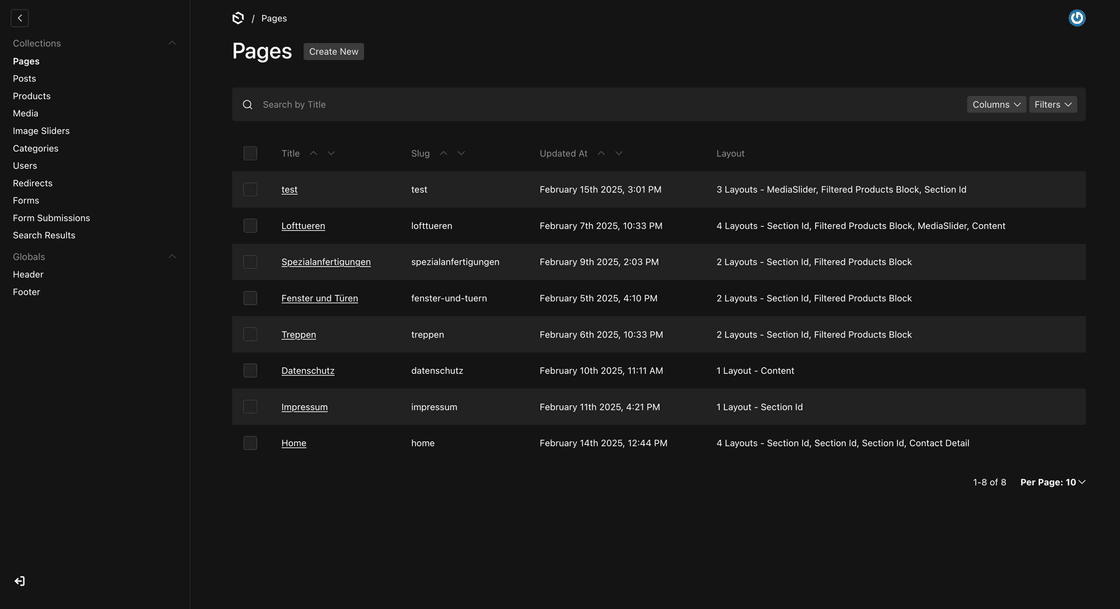
Kausch-Metallbau Website
This is my first project using PayloadCMS.
My colleague Huy Nguyen introduced me to it a few months ago, and I was instantly impressed. It reminded me of the WordPress ACF fields I worked with as a junior developer in my first job.
PayloadCMS it is built with Next.js and TypeScript, seamlessly integrating both frontend and backend within the same repository. The site is a showcase for the company's products and services. It's fully responsive and gives the company the ability to easily manage their content.
Tech Stack Overview
This website is built using a modern and powerful technology stack:
- Next.js: A React framework for production-grade websites
- TypeScript: A statically typed superset of JavaScript
- TailwindCSS: A utility-first CSS framework
- Payload CMS v3: A headless CMS that provides a robust admin interface and API
- MongoDB: A flexible NoSQL database
- AWS S3: A scalable cloud storage solution for media assets
Code Snippets
This is a typical headless CMS implementation where Payload handles the content structure and data storage, while Next.js handles the frontend presentation.
Payload Block Config (Backend Schema)
This block defines a structured content type in Payload CMS with the following features:
Creates a custom block type called "FilteredProductsBlock"
Defines three main fields:
- A title field (text)
- A subtitle field (text)
- A categories field that's an array of relationships to other categories
Allows content editors to select multiple categories through a relationship field
This structure serves as the backend schema definition
import type { Block } from 'payload';
export const FilteredProductsBlock: Block = {
slug: 'filteredProductsBlock',
interfaceName: 'FilteredProductsBlock',
fields: [
{
name: 'filteredProductsTitle',
type: 'text',
required: false,
label: 'Title',
},
{
name: 'filteredProductsSubtitle',
type: 'text',
required: false,
label: 'Subtitle',
},
{
name: 'categories',
type: 'array',
label: 'Categories',
fields: [
{
name: 'category',
type: 'relationship',
relationTo: 'categories',
hasMany: true,
},
],
},
],
};
Payload Block Component (React Implementation)
This is the frontend implementation that:
- Fetches products based on selected categories
- Handles both single categories and arrays of categories
- Processes the data through an API endpoint (/api/products)
- Displays the filtered products in a responsive grid
- Includes title, subtitle, and a back button
- Uses client-side state management to handle the product data
The combination of these two pieces allows content editors to:
- Create filtered product sections in their content
- Select which categories of products to display
- Customize the title and subtitle
- Have the frontend automatically fetch and display the relevant products
This is a typical headless CMS implementation where Payload handles the content structure and data storage, while Next.js handles the frontend presentation.
'use client';
import { BackButton } from '@/components/BackButton';
import { CardProducts } from '@/components/Card/products';
import React, { useEffect, useState } from 'react';
import type { FilteredProductsBlock as FilteredProductsBlockProps } from '../../payload-types';
type Props = FilteredProductsBlockProps & {
showProductsOrPosts?: 'products' | 'posts' | 'filteredProductsBlock';
categories?: any; // support object or string
filteredProductsTitle?: string;
filteredProductsSubtitle?: string;
};
export const FilteredProductsBlock: React.FC<Props> = ({
categories,
filteredProductsTitle,
filteredProductsSubtitle,
}) => {
const [products, setProducts] = useState<any[]>([]);
useEffect(() => {
if (!categories || categories.length === 0) return;
// If categories is an array of objects where each object has a "category" property (an array)
// Otherwise, fallback to assuming the category item itself contains the slug
const catArray = categories.flatMap((item: any) =>
item.category ? item.category : [item]
);
const slugs = catArray.map((cat: any) => cat.slug).filter(Boolean);
const queryParam = slugs.join(',');
// Using "category" query param so that the API endpoint filters products by the provided slugs.
fetch(`/api/products?category=${encodeURIComponent(queryParam)}`)
.then((res) => res.json())
.then((data) => {
setProducts(data.products || []);
});
}, [categories]);
return (
<div className='sm:container mx-auto max-w-7xl px-4 sm:px-6 lg:px-8'>
<div className='flex flex-col items-center text-left'>
{filteredProductsTitle && (
<h2 className='text-3xl font-bold mb-4'>{filteredProductsTitle}</h2>
)}
{filteredProductsSubtitle && (
<p className='text-lg mb-16'>{filteredProductsSubtitle}</p>
)}
<div className='w-full sm:mt-20 mt-6 grid grid-cols-1 lg:grid-cols-2 xl:grid-cols-2 gap-6'>
{products.map((product: any) => (
<CardProducts
key={product.id}
doc={product}
relationTo='products'
/>
))}
</div>
<div className='w-full flex justify-end mt-8'>
<BackButton />
</div>
</div>
</div>
);
};
export default FilteredProductsBlock;